JavaScript this, regular functions, arrow functions : a context.
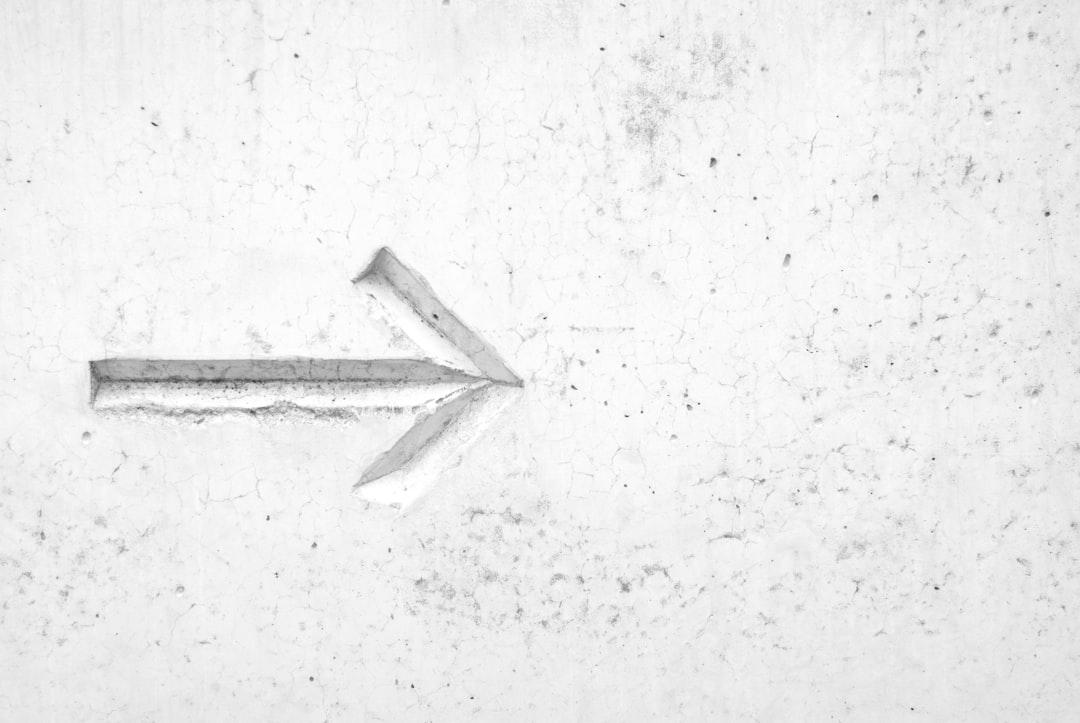
This post will explore the use of arrow functions in JavaScript and how these differ from regular functions and the use of this keyword (this, regular functions, arrow functions).
What is the this keyword ? And how does it works ?
The this keyword is a special keyword that refers to the current object in a function. When you call a function, the this keyword is set to the object that called the function. This means that any variables or functions defined in the calling object are available inside of the function.
In most cases, you don’t need to worry about this keyword. However, there are times when you want to access specific variables or functions from within a function without referencing them from outside of the function. To do this, you can use the this keyword as an alias for the calling object’s variable or function.
The value of the this keyword is based entirely on how it is called. This could be any of the following:
1. A new object
If the function is called with new:
const Boul = new Dog('2 year', 'brown');
The value of this inside the Dog constructor function is a new object because it was called with new.
2. A specified object
If the function is invoked with call/apply:
const check = obj1.checkCompatibility.call(obj2);
The value of this inside checkCompatibility() will refer to obj2 since the first parameter of call() is to explicitly set what this refers to.
3. A context object
If the function is a method of an object:
Dog.bark();
The value of this inside teleport() will refer to data.
4. The global object or undefined
If the function is called without it’s context:
.bark();
When the code above is run, the value of this inside teleport() will either be the global object or undefined if in strict mode.
What are JavaScript regular functions?
JavaScript regular functions are functions that you define in the same way as you would any other function. They take one argument, which is the name of the function itself.
How this works in a JavaScript regular functions?
In a regular function, the this keyword refers to the current object. This means that if you call a regular function from within another function, the this keyword will refer to the outermost function.
What are JavaScript arrow functions?
Arrow functions are a new feature of the language that allow you to define functions as anonymous objects.
Arrow functions are a new addition to the JavaScript language and can be used to simplify code. They work by taking a single parameter, which is usually a function, and returning a new function that takes the same parameter. This means that arrow functions can be used to create short, concise code.
However, arrow functions cannot be used in the same way as regular functions. For example, they cannot be called from within another arrow function or from within a script block. This means that you need to use the this keyword when calling an arrow function from elsewhere in your code.
Arrow functions are a new type of function in JavaScript. They’re similar to regular functions, but they have one key difference: they use the this keyword to reference the current object. This makes arrow functions perfect for working with objects that you want to use as context in your code.
How do arrow functions work?
Arrow functions are a new way of writing code in JavaScript. They work a bit differently than regular functions. Here’s how they work:
When you call an arrow function, you first provide the name of the function. Then, you provide the arguments that will be passed to the function. Finally, you call the function.
The this keyword refers to the context in which the arrow function was called. This means that any variables or properties that were defined when the arrow function was called will still be available when it executes.
Why use arrow functions?
Arrow functions are a new type of function in JavaScript that allow you to write concise and readable code. Arrow functions are especially useful when you need to perform multiple operations on the same data. For example, you can use an arrow function to calculate the sum of two arrays, or to find the index of a element in an array.
Examples of arrow functions and this
Arrow functions are a newer feature of JavaScript that allow you to write shorter, more concise code. They’re similar to regular functions, but they have a fat arrow function syntax. Here’s an example:
var myArrowFunction = function(x) { return x * 2; };
This code creates a new function called myArrowFunction that takes one parameter, x. The this keyword refers to the current object instance (in this case, the document). The return statement returns x * 2, which is the value of x multiplied by 2. Arrow functions are useful for creating short, reusable code blocks.
Let’s see this, regular functions and arrow functions in action
Conclusion: this, regular functions, arrow functions.
The value of this inside an arrow function is the same as the value of this outside the function.
Arrow functions are a powerful new feature of the JavaScript language. They allow you to define Functions as anonymous objects, which can lead to cleaner and more concise code.